A linked list can be considered as a train, where each boogie is connected by links. Formally defined, a linked list is a linear data structure that consists of nodes that are connected. Each node has some data associated with it, along with the address of the next node. The last node of a linked list points to NULL.
Takeaways
The linked list is a linear data structure that has some data associated with them and consists of nodes that are connected, with the next pointer.
For What Applications a Linked List can be Used ?
Before getting started with what applications a Linked List can be used, let us first know what are linked lists.
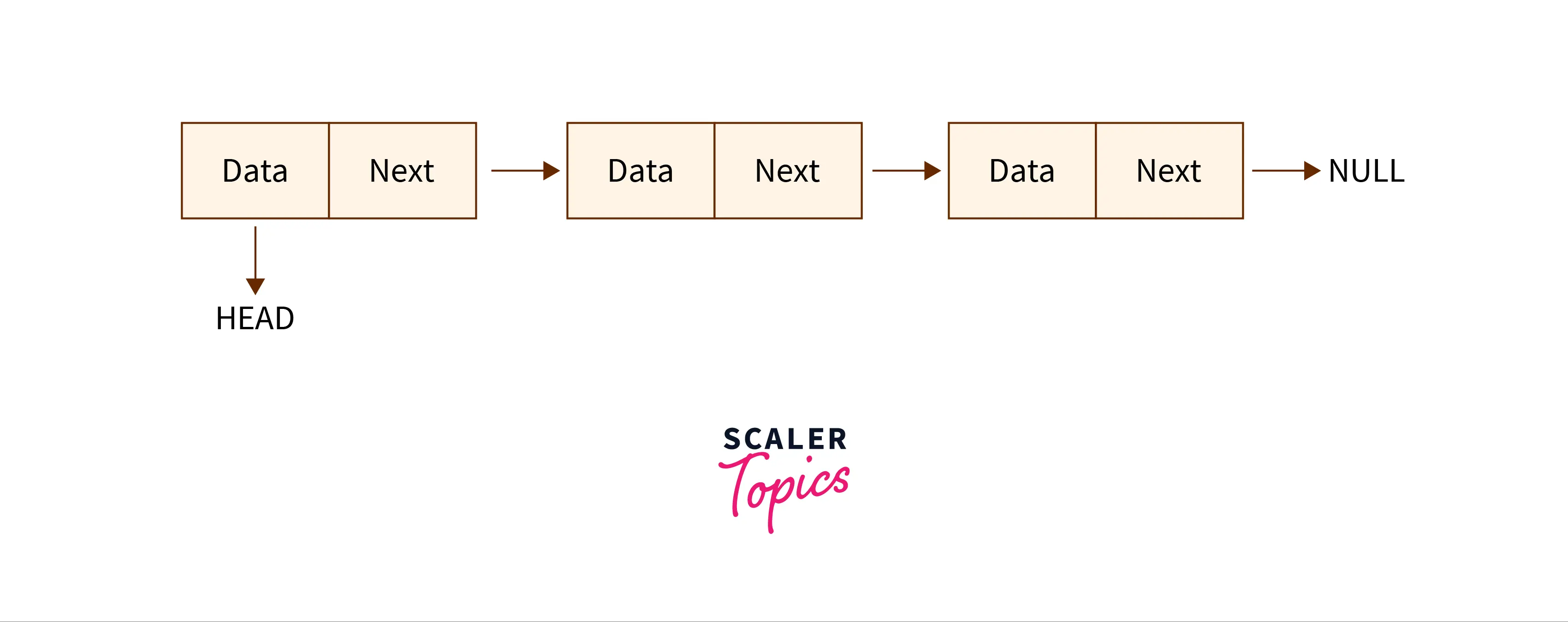
There are numerous applications of linked lists. In this article, we are going to discuss What Application a Linked List Can Be Used. Now that we have covered the basics of linked lists, let us get started with the applications of the same.
For What Application a Linked List can be Used in Computer Science ?
Linked lists are widely used in computer science for the range of advantages they provide. Let us look down at some of the popular applications of the linked list in computer science.
1. Implementing Advanced Data Structures Using Linked Lists
Data structures like stacks and queues can be implemented using linked lists.
But how to implement stack using linked list ?
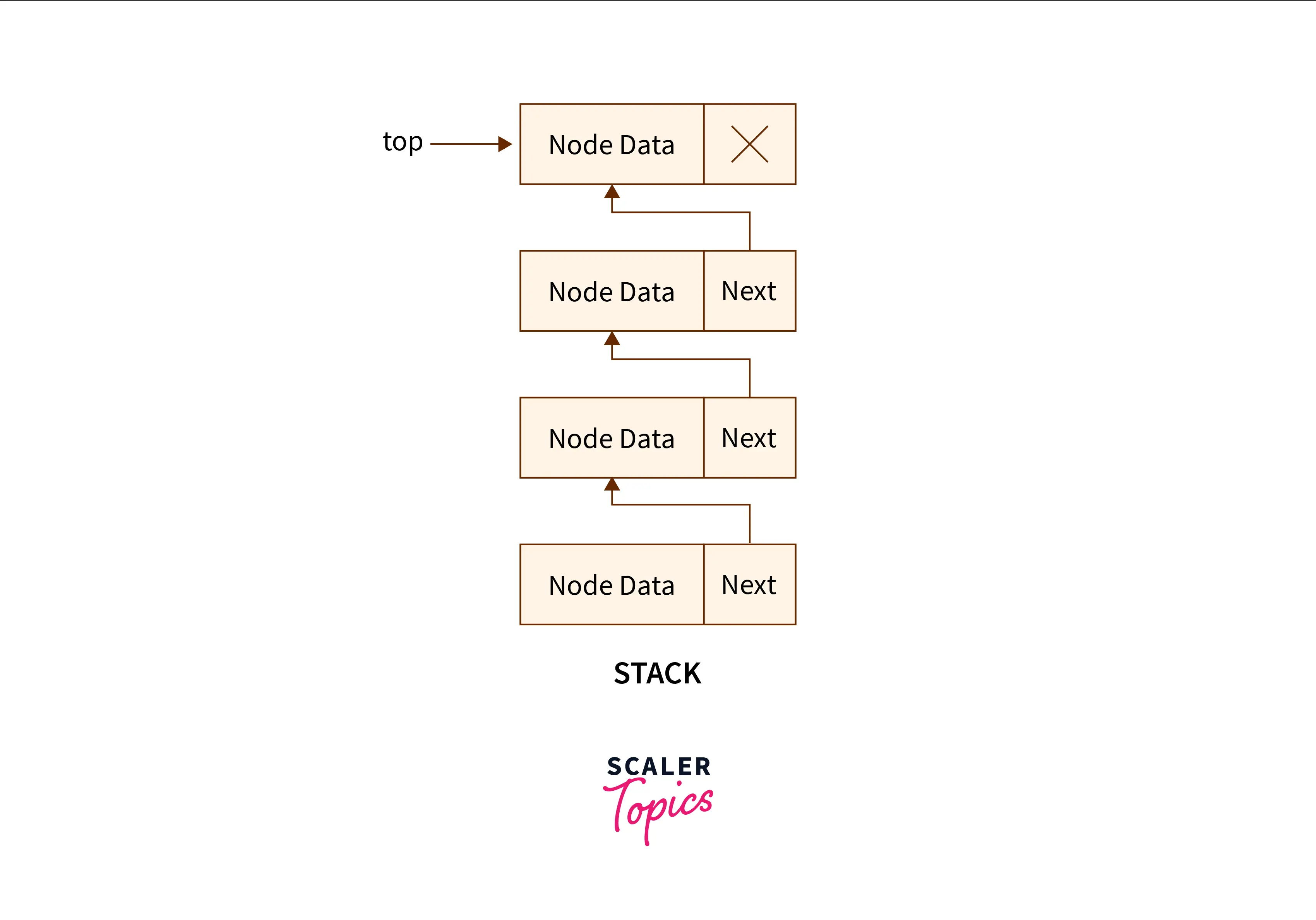
- The head of the stack (top) is the point where the pushing and popping of items take place.
- That head can be considered the head of the linked list.
- The first node of the stack (implemented using a linked list) has Null in the link field. The next node’s link field points to the head and consecutive nodes point to the node before them.
But why use a linked list over an array to implement stack/queue ?
The primary reason that can be considered to do so is that we can have a dynamic stack or queue, whose length we can alter as per our requirement. We can shrink or grow our linked list, there are no restrictions associated. However, with an array, there is a fixed size.
2. Graph Adjacency List Representation Using Linked Lists
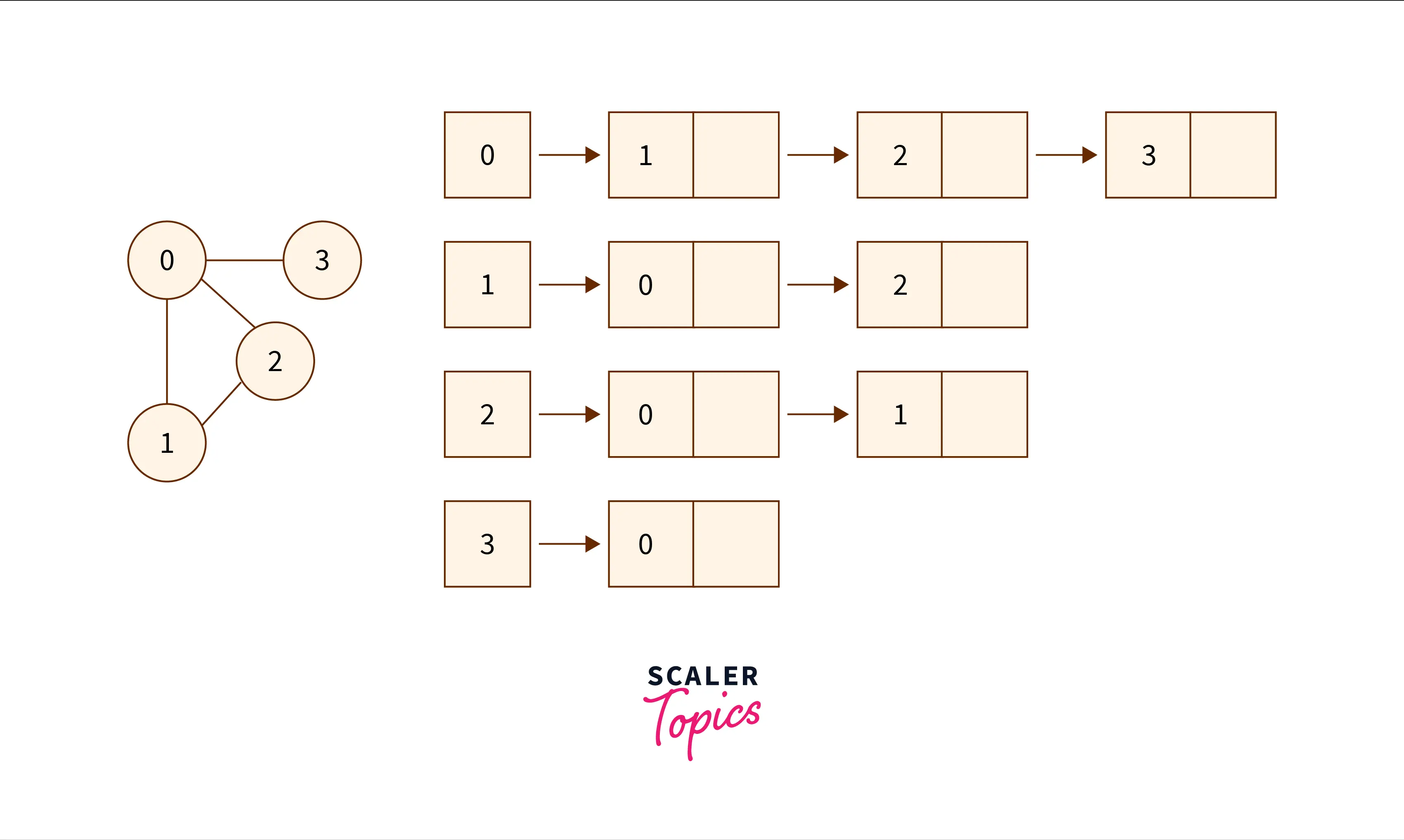
Adjacency list is one of the representations of the Graph data structure. The adjacency list represents a graph as an array of linked lists. The index of the array in the adjacency list represents a vertex of the graph and each element in its linked list represents the other vertices that form an edge with the vertex of the graph.
3. Dynamic Memory Allocation Using Linked Lists
Linked lists are always preferable when we are not sure about the amount of memory we may end up using for storing data. In that case, if we use an array then there are chances when we might need more memory, thereby creating new arrays with increased size. This will add a lot of overhead to the system. Hence, linked lists are always preferred in those cases because we can dynamically allocate memory in linked lists.
4. Arithmetic Operations Using Linked Lists for Long Integers
In most programming languages, there is a limitation associated with the value that integers can store. Also, we usually cannot perform arithmetic operations on the long integers because of the overflow error which we might get. However, using a linked list to represent the long integers proves to be beneficial here, because we can then perform these arithmetic operations.
5. Manipulating Polynomials Using Linked Lists
Linked lists can be used to execute polynomial manipulations like addition, subtraction, and differentiation, among them. Linked lists can be used to represent polynomials and the different operations that can be performed on them.
For example the polynomial $6x^3 + 9x^2 + 7x + 1$ can be represented by a linked list as below :
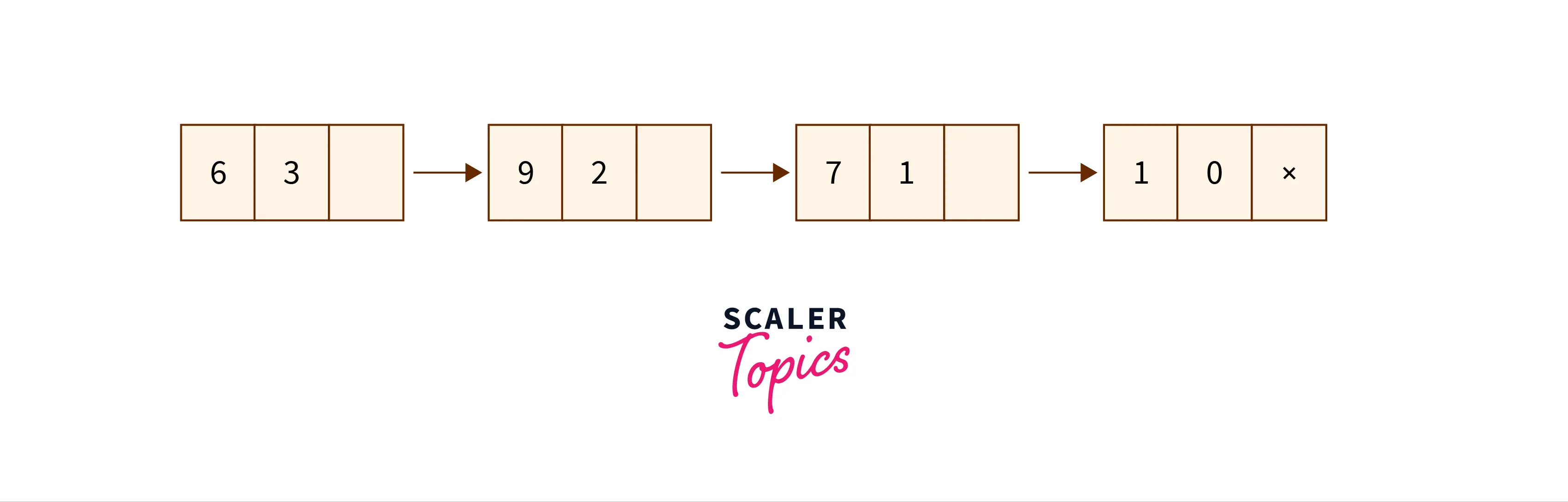
For What Application a Linked List can be used in the Real World ?
There are multiple applications of linked lists in the real world. Let us look at a few of them below.
1. Linked List in Web Browsers
You might have noticed that the back and forward buttons in web browsers can always be used to view the previous and next URL. The main reason for this is that they are connected using a linked list, hence access to the previous and subsequent URLs searched is made possible.
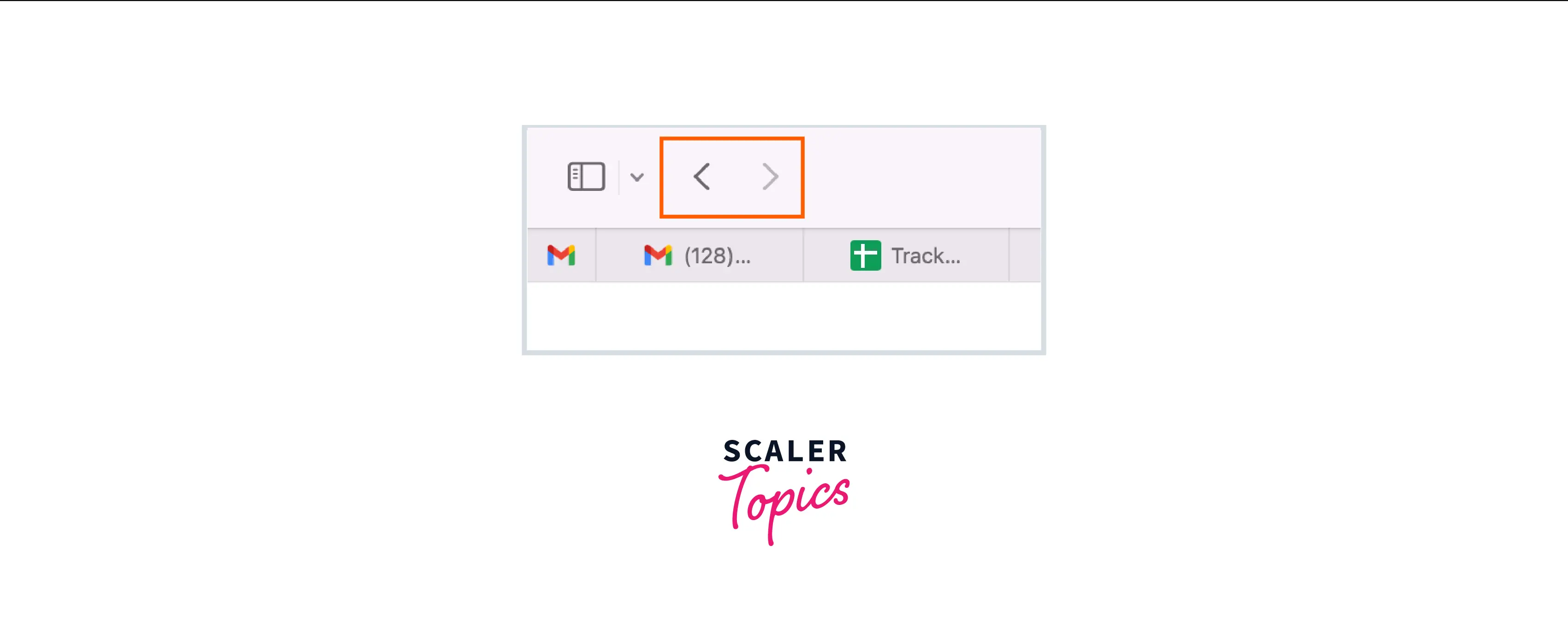
2. Linked List in Music Players
Just like we discussed in the case of web browsers, the songs in the Music Player too are linked to the next and the previous songs. We can play any song from either the starting or the ending of the list. They all are linked through the linked lists.
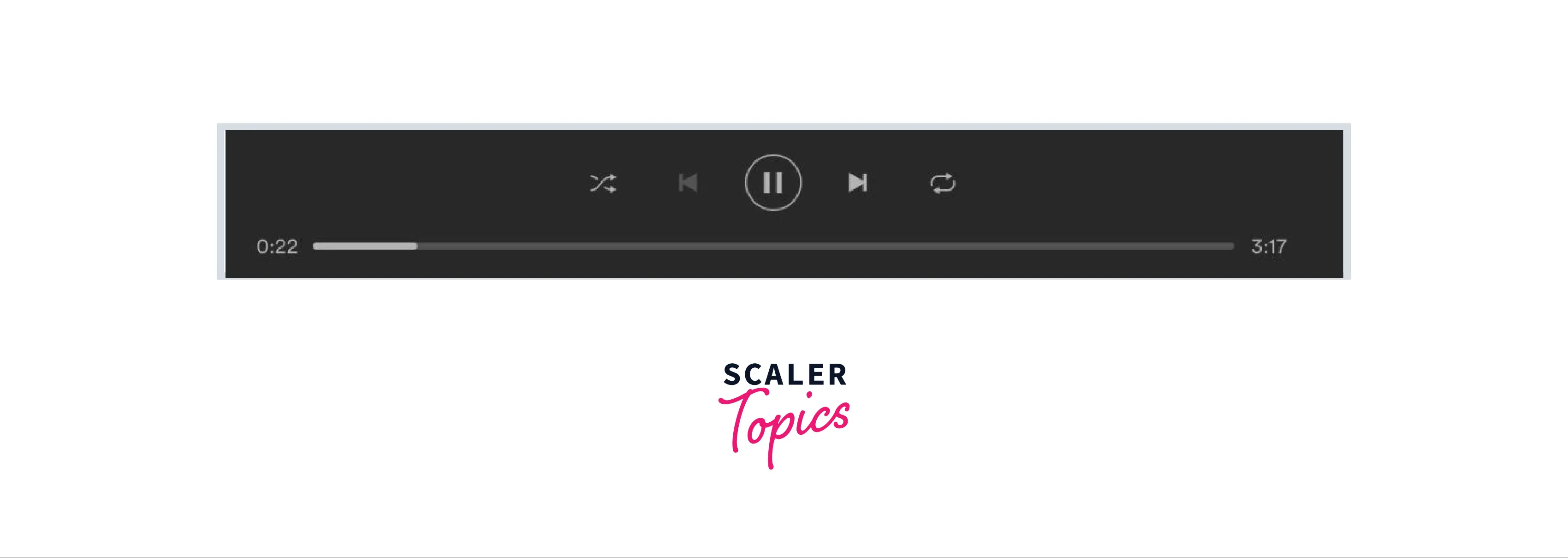
3. Linked List in Image Viewers
Similar to our previous examples, you might have also seen there are next and previous options in the image viewers. We can access the previous and the next images using the buttons. They are again linked through linked lists.
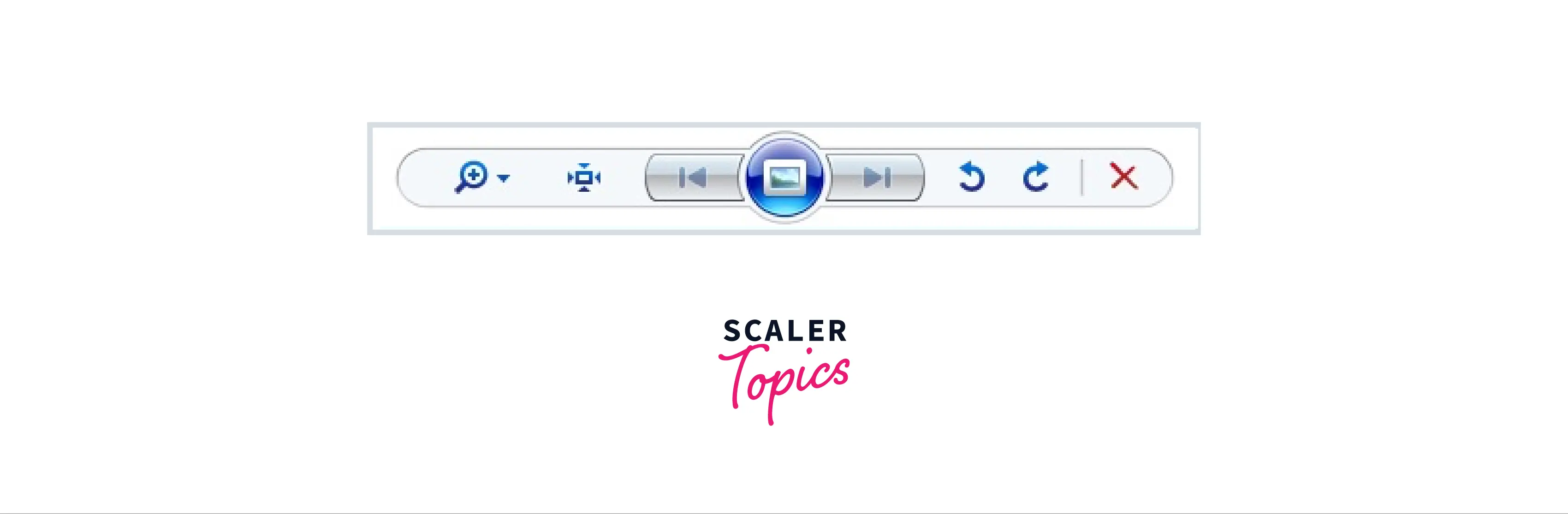
4. Operating System
Linked lists are used in the time-sharing problem, which is used by the scheduler during the scheduling of any processes in the Operating system.
For What Application a Linked List can be Used in Circular Linked List ?
A circular linked list is a type of linked list, where the first and last nodes are connected and form a kind of circle.
Let us look at the applications of the circular linked lists.
- Circular linked lists can be used to implement the advanced type of data structures like the Fibonacci heaps.
- Usually, when multiple programs are running on a machine, the operating system typically lists all of them into a list, and then cycles over them. By doing so, it gives each of the programs a certain time to execute before having them wait while the CPU is given to another. In that case, the operating system usually uses a circular linked list for cycling over the processes. In the same, it can cycle back to the beginning whenever it reaches the end of all programs.
- Implementing a queue can be done using circular linked lists. If we utilize a circular linked list, we do not have to keep track of the head and rear pointers. The head can always be found as the next node in the list, and we can store a pointer to the list’s last node.
For What Application can a Doubly Circular Linked List be Used ?
In a circular doubly linked list, two consecutive elements are linked or connected by a previous and next pointer, and the last pointer points to the first pointer, as well as the first pointer points to the last pointer.
Below given are the advantages of the doubly linked lists over the single linked lists.
- Since the Doubly linked lists have the next and previous pointers, it supports traversing in both directions as opposed to the singly linked list, which only supports traversing in one direction.
- A doubly linked list makes it simpler to remove (delete) nodes than a singly linked list. This is because, to delete a node, we also need access to the node that came before it. Therefore, in a singly linked list, two pointers are needed for deletion, whereas in a doubly linked list, each node already contains the information of the previous node, eliminating the need for an additional pointer.
- A doubly linked list is also simple to reverse. A doubly-linked list can be reversed by simply switching the next and previous pointers for each node and updating the head node to point to the last node.
Few applications for doubly linked lists are :
- In navigation systems that need to perform both forward and reverse navigation, a doubly linked list is used.
- It is used to construct the most recently used (MRU) and least recently used (LRU) cache.
- Undo Redo operations can also be performed using a Doubly Linked list.
- The representation of a traditional game deck of cards can also be done using a doubly linked list.
Advantages of Linked List over Arrays
Every data structure has its own set of pros and cons. Similarly, Linked Lists and arrays have both advantages and disadvantages. But in this topic, we will learn, when and why we prefer a linked list over arrays. Below given are the advantages of the linked list over arrays.
Advantages of Linked List :
- Dynamic Data Structure :
A linked list does not require an initial size because it is a dynamic data structure that can change in size at runtime by releasing or allocating memory. In contrast, an array requires the declaration of a beginning size and a maximum number of elements that cannot be exceeded. Hence we always prefer a linked list over an array whenever we think that there are huge possibilities for our data to grow or shrink in size. - Implementation of other data structures :
A Linked List makes it simple to create certain practical data structures like queues and stacks, also a doubly linked list can be used for the implementation of the Fibonacci heaps. The LRU Cache too can be implemented using a doubly linked list. - Insertion and Deletion Operation :
As there is no need to shift every member after insertion or deletion in a Linked List, these operations are fairly simple as compared to that of arrays. In linked lists, only the address contained in the pointers needs to be changed. whereas we need to shift elements within an array. - No Memory Wastage :
In the linked list there is very less memory waste(due to storing the address of the next pointers) or no memory wastage because a linked list’s size can change as needed. Memory is only allocated whenever needed. Whereas in arrays, we must initialize it with a size that we may or may not use completely, resulting in memory waste.
Learn More About Linked List in Data Structures
Now that we have covered “For What Applications a Linked List Can Be Used” in detail, I encourage you to go ahead and pick the below scaler articles to learn more about linked lists.
Conclusion
In this article, we learned about “For What Application a Linked List Can Be Used”. Let us get a quick recap of what we learned throughout.
- We can implement advanced data structures like the stack, and queue with linked lists. Also, the adjacency list for graphs and the LRU cache can be implemented using it. Also, the forward and backward buttons in web browsers are implemented using linked lists.
- Linked lists can be used in the music player systems, image viewers, etc.
- Circular linked lists are also very useful for the implementation of Fibonacci heaps and are also used by the OS to allocate CPU time to processes.
- Doubly linked lists can be used for navigation operations, undo, redo operations, etc.
- The biggest advantage of the linked list over arrays lies in the dynamic memory allocation. Also, no memory wastage takes place in the linked lists.