This exercise will demonstrate how to obtain the sum of cubes of n
natural numbers. Here, a single for loop from 1
to n
is being used. We compute the term’s cube in each step before adding it to the total. This program executes in O(n)
time. However, we may use this series formula given below to solve it in O(1)
or constant time.
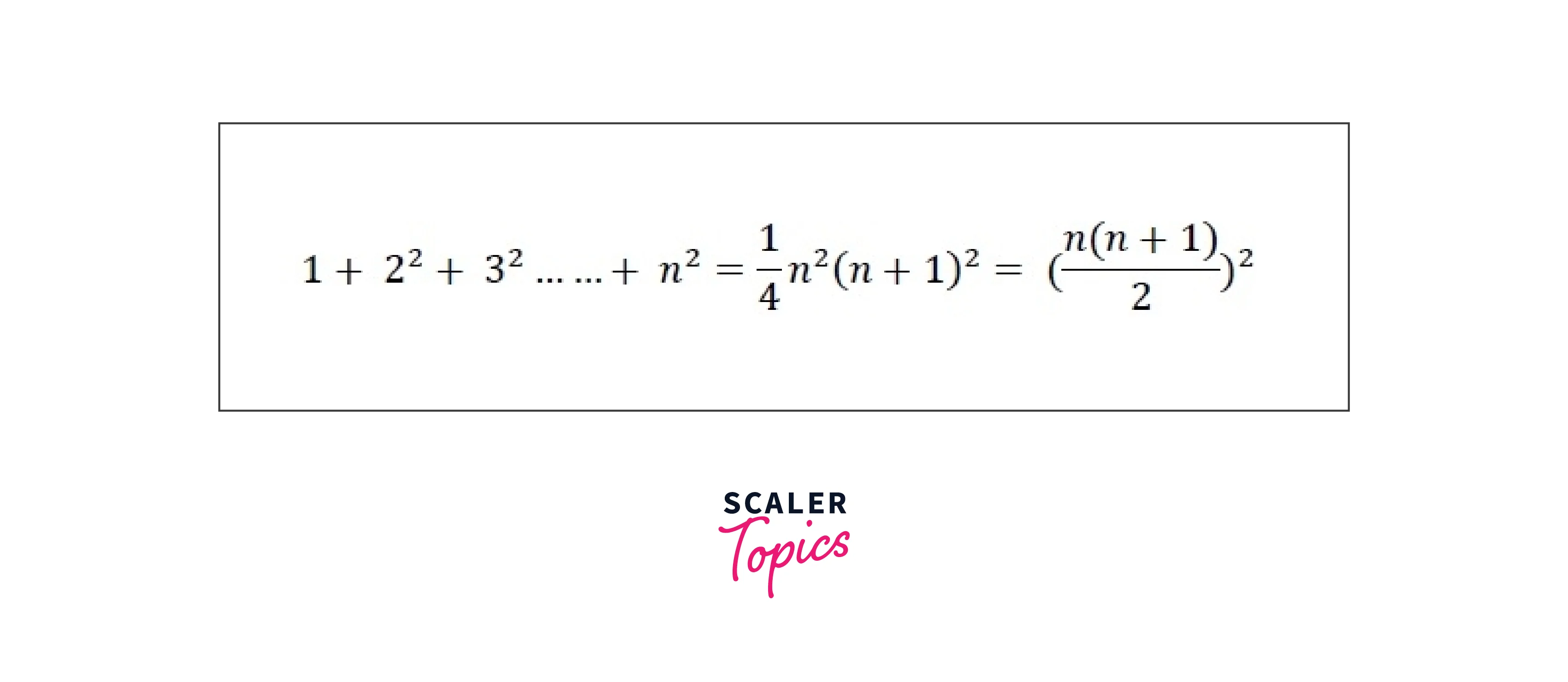
The formula for the sum of cubes of the first n even natural numbers is:
$$
Sum = n(n + 1)(2n + 1)/6
$$
The formula for the sum of cubes of the first n odd natural numbers is:
$$
Sum = n^2 * (n^2 – 1)
$$
The Sum of the Cube of First n Natural Numbers
Print the sum of series $1^3 + 2^3 + 3^3 + 4^3 + …….+ n^3$ till n-th
term.
Example
Examples of the series are as follows:
Input: n = 2
Output: 9
$$
1^3 + 2^3 = 9
$$
Input: n = 5
Output: 225
$$
1^3 + 2^3 + 3^3 + 4^3 + 5^3 = 225
$$
Solution:
A simple solution is to add terms one by one.
Implementation
Adding Terms One by One
Simple C++ program to find the sum of series with cubes of first n natural numbers:
- Initialize a variable to store the sum and iterate from
1
ton
, adding the cube of each natural number to the sum in each iteration. - After the loop finishes, the sum will contain the result of the series sum of the cubes of the first
n
natural numbers.
#include <iostream>
using namespace std;
/* This function returns the sum of series */
int sumOfTheSeries(int num)
{
int sum = 0;
for (int i = 1; i <= num; i++)
sum += i * i * i;
return sum;
}
// Driver Function
int main()
{
int num = 5;
cout << sumOfTheSeries(num);
return 0;
}
Simple Java program to find the sum of series with cubes of first n natural numbers:
import java.util.*;
import java.lang.*;
class SumSeries{
/* Returns the sum of series */
public static int sumOfTheSeries(int num)
{
int sum = 0;
for (int i = 1; i <= num; i++)
sum += i * i * i;
return sum;
}
// Driver Function
public static void main(String[] args)
{
int num = 5;
System.out.println(sumOfTheSeries(num));
}
}
Simple Python program to find the sum of series with cubes of first n natural numbers:
# Returns the sum of the series
def sumOfTheSeries(num):
sum = 0
for i in range(1, num + 1):
sum += i * i*i
return sum
# Driver Function
n = 5
print(sumOfTheSeries(num))
Output:
225
- Time Complexity:
O(n)
- Auxiliary Space:
O(1)
Using Formula
The direct mathematical formula
$$
(n (n + 1) / 2)
$$2
is an effective way to solve the problem.
For n = 5 sum by formula is:
$$
(5(5 + 1 ) / 2)) ^ 2 = (56/2) ^ 2
= (15) ^ 2
= 225
$$
For n = 7, sum by formula is:
$$
(7(7 + 1 ) / 2)) ^ 2 = (78/2) ^ 2
= (28) ^ 2
= 784
$$
A formula-based C++ program to find the sum of a series with cubes of first n natural numbers:
#include <iostream>
using namespace std;
int sumOfTheSeries(int num)
{
int i = (num * (num + 1) / 2);
return i * i;
}
// Driver Function
int main()
{
int num = 5;
cout << sumOfTheSeries(num);
return 0;
}
A formula-based Java program to find the sum of series with cubes of first n natural numbers:
import java.util.*;
import java.lang.*;
class SumSeries {
/* Returns the sum of series */
public static int sumOfTheSeries(int num)
{
int i = (num * (num + 1) / 2);
return i * i;
}
// Driver Function
public static void main(String[] args)
{
int num = 5;
System.out.println(sumOfTheSeries(num));
}
}
A formula-based Python program to find the sum of series with cubes of first n natural numbers:
# Returns the sum of series
def sumOfTheSeries(num):
i = (num * (num + 1) / 2)
return (int)(i * i)
# Driver Function
num = 5
print(sumOfTheSeries(num))
Output:
225
- Time Complexity:
O(1)
- Auxiliary Space:
O(1)
Program to Find Sum of Cube of N Even Natural Numbers
Find the Sum of the First n Even Natural Numbers of Given n.
Examples:
Input: 2
Output: 72
$$
2^3 + 4^3 = 72
$$
Input: 8
Output: 10368
$$
2^3 + 4^3 + 6^3 + 8^3 + 10^3 + 12^3 + 14^3 + 16^3 = 10368
$$
Finding the sum of the cubes by iterating through n even numbers is easy.
Simple C++ method to find the sum of cubes of first n even numbers:
#include <iostream>
using namespace std;
int cubeSum(int num)
{
int sum = 0;
for (int i = 1; i <= num; i++)
sum += (2*i) * (2*i) * (2*i);
return sum;
}
int main()
{
cout << cubeSum(8);
return 0;
}
Java program to perform sum of cubes of first n even natural numbers:
public class CubeSum
{
public static int cubesum(int num)
{
int sum = 0;
for(int i = 1; i <= num; i++)
sum += (2 * i) * (2 * i)
* (2 * i);
return sum;
}
// Driver function
public static void main(String args[])
{
int num = 8;
System.out.println(cubesum(num));
}
}
Python3 program to find the sum of cubes of first n even numbers:
# Function to find the sum of cubes
# of first n even numbers
def cubeSum(num):
sum = 0
for i in range(1, num + 1):
sum += (2 * i) * (2 * i) * (2 * i)
return sum
# Driven code
print(cubeSum(8))
Output
10368
- Time Complexity:
O(n)
- Auxiliary Space:
O(1)
To solve the problem effectively, use the formula below.
sum = 2 * n2(n+1)2
We are aware that $$
n2(n+1)2 / 4$$
is the sum of the cubes of the first n
natural numbers.
Sum of cubes of first n natural numbers=
$$
2^3 + 4^3 + …. + (2n)^3
= 8 * (1^3 + 2^3 + …. + n^3)
= 8 * n2(n+1)2 / 4
= 2 * n2(n+1)2
$$
Example
Efficient C++ method to find the sum of cubes of first n even numbers:
#include <iostream>
using namespace std;
int cubeSum(int num)
{
return 2 * num * num * (num + 1) * (num + 1);
}
int main()
{
cout << cubeSum(8);
return 0;
}
Java program to perform sum of cubes of first n even natural numbers:
public class EvenNatural
{
public static int cubesum(int num)
{
return 2 * num * num * (num + 1) * (num + 1);
}
// Driver function
public static void main(String args[])
{
int num = 8;
System.out.println(cubesum(num));
}
}
Python3 program to find the sum of cubes of first n even numbers:
# Function to find the sum of cubes
# of first n even numbers
def cubeSum(num):
return 2 * num * num * (num + 1) * (num + 1)
# Driven code
print(cubeSum(8))
Output:
10368
- Time Complexity:
O(1)
- Auxiliary Space:
O(1)
Program to Find Sum of Cube of N Odd Natural Numbers
Find the Sum of the First n Odd Natural Numbers Given n.
Input: 2
Output: 28
$$
1^3 + 3^3 = 28
$$
Input: 4
Output: 496
$$
1^3 + 3^3 + 5^3 + 7^3 = 496
$$
Finding the sum of the cubes by iterating through n odd numbers is an easy solution.
Simple C++ method to find the sum of cubes of first n odd numbers:
#include <iostream>
using namespace std;
int cubeSum(int num)
{
int sum = 0;
for (int i = 0; i < num; i++)
sum += (2*i + 1)*(2*i + 1)*(2*i + 1);
return sum;
}
int main()
{
cout << cubeSum(2);
return 0;
}
Java program to perform sum of cubes of first n odd natural numbers:
public class CubeOdd
{
public static int cubesum(int num)
{
int sum = 0;
for(int i = 0; i < num; i++)
sum += (2 * i + 1) * (2 * i +1)
* (2 * i + 1);
return sum;
}
// Driver function
public static void main(String args[])
{
int z = 5;
System.out.println(cubesum(z));
}
}
Python3 program to find sum of cubes of first n odd numbers:
def cubeSum(num):
sum = 0
for i in range(0, num) :
sum += (2 * i + 1) * (2 * i + 1) * (2 * i + 1)
return sum
# Driven code
print(cubeSum(2))
C# program to perform sum of cubes of first n odd natural numbers:
using System;
public class FirstOdd
{
public static int cubesum(int num)
{
int sum = 0;
for(int i = 0; i < num; i++)
sum += (2 * i + 1) * (2 * i +1)
* (2 * i + 1);
return sum;
}
// Driver function
public static void Main()
{
int a = 5;
Console.WriteLine(cubesum(a));
}
}
Output
28
Complexity Analysis
Time Complexity: O(n)
, since the cubeSum()
function only does one traverse.
Space Complexity: O(1)
Applying the formula below will result in an effective solution.
$$
sum = n^2 (2n^2 – 1)
$$
How Does It Function?
We are aware that
$$
n2(n+1)2 / 4
$$
is the sum of the cubes of the first n
natural numbers.
Sum of cubes of first n
odd
natural numbers =
Sum of cubes of first 2n
natural numbers –
Sum of cubes of first n
even
natural numbers
$$
= (2n)2(2n+1)2 / 4 – 2 * n2(n+1)2
= n2(2n+1)2 – 2 * n2(n+1)2
= n2[(2n+1)2 – 2*(n+1)2]
= n2(2n2 – 1)
$$
Efficient C++ method to find sum of cubes of first n odd numbers:
#include <iostream>
using namespace std;
int cubeSum(int num)
{
return num * num * (2 * num * num - 1);
}
int main()
{
cout << cubeSum(4);
return 0;
}
Java program to perform sum of cubes of first n odd natural numbers:
public class FirstOdd
{
public static int cubesum(int num)
{
return (num) * (num) * (2 * num * num - 1);
}
// Driver function
public static void main(String args[])
{
int a = 4;
System.out.println(cubesum(a));
}
}
Python3 program to find the sum of cubes of first n odd numbers:
# Function to find the sum of cubes
# of first n odd number
def cubeSum(num):
return (num * num * (2 * num * num - 1))
# Driven code
print(cubeSum(4))
Output:
496
Complexity Analysis
- Time Complexity:
O(1)
- Space Complexity:
O(1)
FAQs
Q. What is the sum of the first n natural number cubes?
A. The counting of numbers that begin with 1 and proceed to infinity is known as natural numbers. Finding the sum of the cubes of the first n natural numbers involves combining the cubes of a given number of natural integers beginning with 1. For instance, you can write the sum of the cubes of the first five natural numbers as
$$
13 + 23 + 33 + 43 + 53
$$
, the first ten natural numbers as
$$
13 + 23 + 33 + 43 + 53 + 63 + 73 + 83 + 93 + 103
$$
, and so on.
Q. What is the formula for the sum of cubes of n natural numbers?
A. The formula for the sum of cubes of n natural integers is as follows:
The formula to calculate the sum is,
$$
13 + 23 + 33 + 43 +… + n3
$$
if we have a series of cubes of n natural numbers.
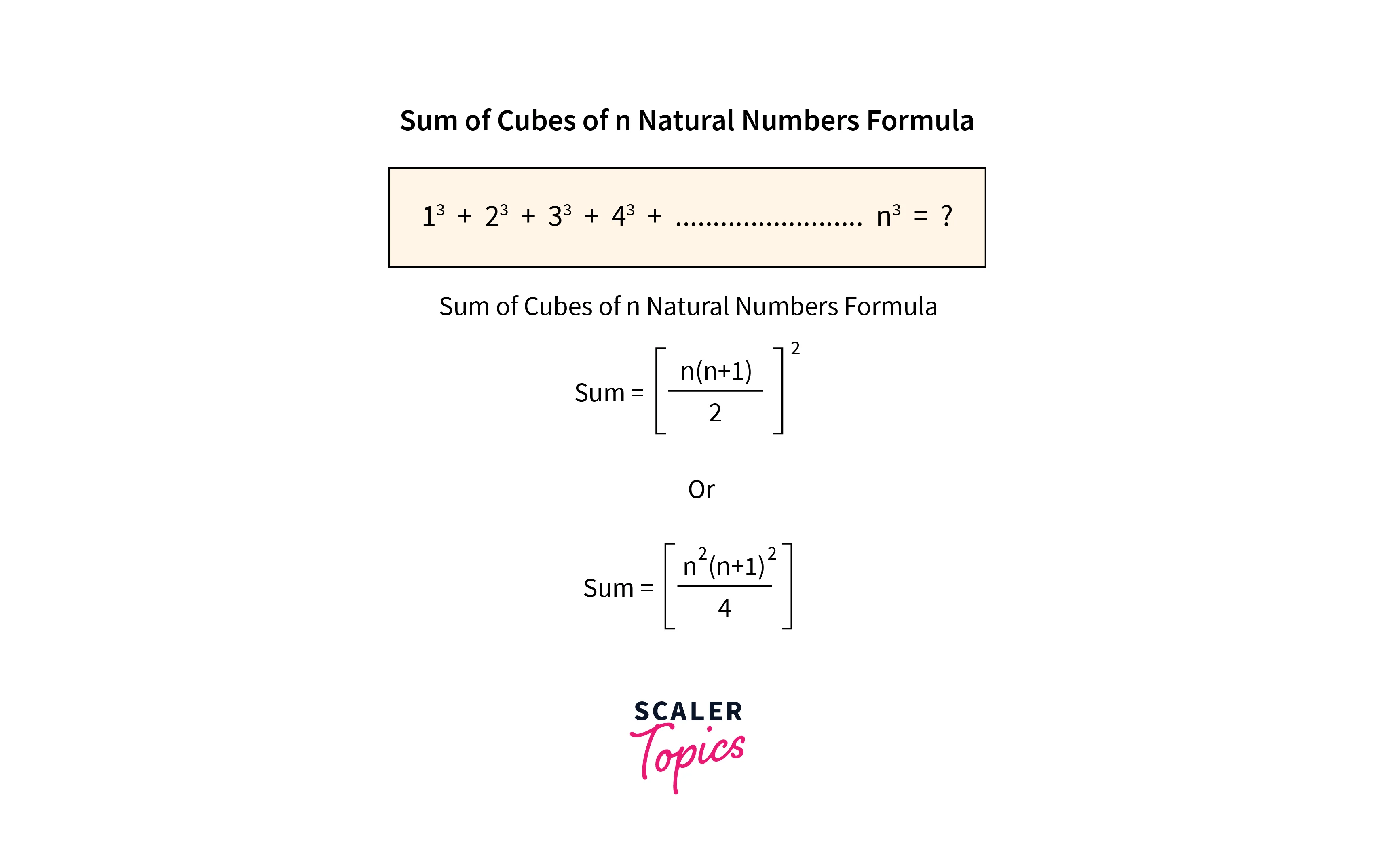
where n is the total number of natural numbers, counting from 1, that have been used.
Q. Pseudo code or steps calculating the sum of cubes of n natural numbers?
A. We will use a simple iterative method in which any loop, such as a for-loop, while-loop, or do-while loop, may be used:
- From 1 to n times, iterate i.
- For every i find it’s a cube.
- Continue to add each cube to the sum variable.
- Return the sum variable.
- Print the result.
Conclusion
- A program to find the sum of cubes of n natural numbers is a common computational task that involves summing the cubes of integers from
1
ton
. - It can be implemented using a simple loop structure, typically a
for
orwhile
loop, to iterate through the natural numbers from 1 ton
. - For each natural number in the range, its cube is calculated and added to a running sum variable, which stores the cumulative sum of cubes.
- The loop continues until all natural numbers from
1
ton
have been processed, resulting in the sum of the cubes. - Efficient algorithms can optimize this calculation by using the formula for the sum of cubes, which reduces the time complexity from
O(n)
toO(1)
for finding the sum of cubes of the first n natural numbers.